Hashing (also known as hash functions) in cryptography is a process of mapping a binary string of an arbitrary length to a small binary string of a fixed length, known as a hash value, a hash code, or a hash.
Hash functions are a common way to protect secure sensitive data such as passwords. Some of the modern commonly-used hash functions are MD5, RIPEMD160, SHA1, SHA256, SHA384, and SHA512.
Hashing is a one-way conversion. You cannot un-hash hashed data. Hashing is used in blockchain to compare and validate the data authenticity. Hashing creates a digital figure print of a file also known as checksums that can be used to verify that a file has not been tempered with.
For example, the following text is converted to 64 character hash.
Now, if you change data to the text block, the Hash will always be a 64-character string.
Compute SHA256 Hash in C#
Hashing (also known as hash functions) in cryptography is a process of mapping binary string of an arbitrary length to a small binary string of a fixed length, known as a hash value, a hash code, or a hash. Hash functions are a common way to protect secure sensitive data such as passwords and digital signatures. Some of the modern commonly used hash functions are MD5, RIPEMD160, SHA1, SHA256, SHA384, and SHA512.
Hashing is a one-way conversion. You cannot un-hash hashed data.
The NET framework provides cryptography related functionality encapsulated in System.Security.Cryptography namespace and its classes. The HashAlgorithm class is the base class for has algorithms including MD5, RIPEMD160, SHA1, SHA256, SHA384, and SHA512.
The ComputeHash method of HashAlgorithm computes a hash. It takes a byte array or stream as an input and returns a hash in form of a byte array of 256 chars.
- byte[] bytes = sha256Hash.ComputeHash(Encoding.UTF8.GetBytes(rawData));
No matter how big the input data is, the hash will always be 256 chars. The following code snippet is an example of how to create a hash of a string.
- using System;
- using System.Text;
- using System.Security.Cryptography;
-
- namespace HashConsoleApp
- {
- class Program
- {
- static void Main(string[] args)
- {
- string plainData = "Mahesh";
- Console.WriteLine("Raw data: {0}", plainData);
- string hashedData = ComputeSha256Hash(plainData);
- Console.WriteLine("Hash {0}", hashedData);
- Console.WriteLine(ComputeSha256Hash("Mahesh"));
- Console.ReadLine();
- }
-
- static string ComputeSha256Hash(string rawData)
- {
-
- using (SHA256 sha256Hash = SHA256.Create())
- {
-
- byte[] bytes = sha256Hash.ComputeHash(Encoding.UTF8.GetBytes(rawData));
-
-
- StringBuilder builder = new StringBuilder();
- for (int i = 0; i < bytes.Length; i++)
- {
- builder.Append(bytes[i].ToString("x2"));
- }
- return builder.ToString();
- }
- }
-
- }
- }
Blockchain and hashing
In Blockchain technology, hashing is used to stop data tempering and manipulation.
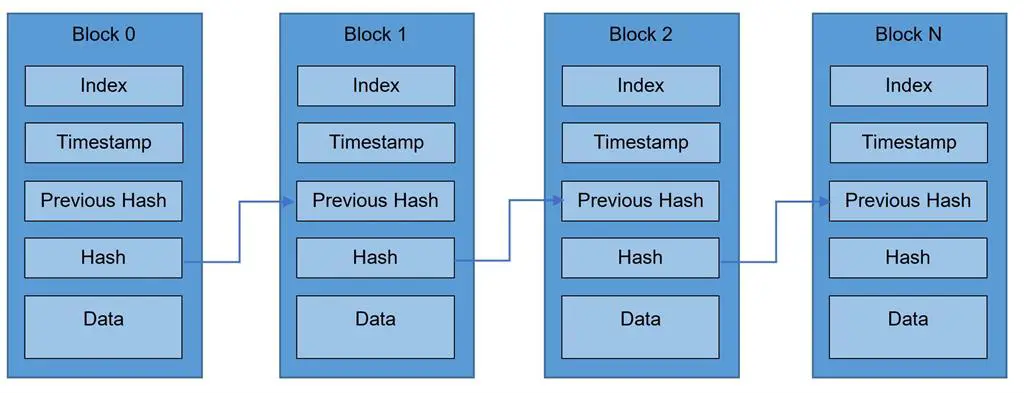
Before a new block is approved and added to a blockchain, the block data is hashed. Each new block has a reference to the previous block's hash and if anything changes in a block, the hash value is changed and the block is no longer valid. This is useful when hackers try to add a block that is not approved by the network.